使用 Vue3 Suspense 优雅处理异步组件加载
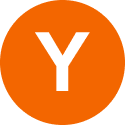
YGHub·2024-11-19·2·字数:403 字·阅读时间:2 分钟
在开发中,我们经常需要处理异步数据加载的场景。今天就来聊聊 Vue3 中的 “救星” —— Suspense
组件,看看它是如何帮我们优雅地解决这些问题的。
一、Suspense
是什么?
简单来说,Suspense
就像是一个"等待室",可以优雅地处理异步组件和异步数据:
- 在数据加载时显示
loading
状态 - 加载完成后自动显示实际内容
- 加载失败时显示错误提示
二、基本使用方法
1. 最简单的例子
vue
<template> <Suspense> <!-- 默认插槽:放置异步组件 --> <template #default> <AsyncComponent /> </template> <!-- fallback插槽:加载状态 --> <template #fallback> <div>Loading...</div> </template> </Suspense></template> <script setup>// 异步组件const AsyncComponent = defineAsyncComponent(() => import('./components/AsyncComponent.vue'))</script>
2. 处理异步数据
vue
<!-- AsyncComponent.vue --><template> <div> <h1>{{ data.title }}</h1> <p>{{ data.content }}</p> </div></template> <script setup>// 使用 async setupconst data = await fetch('https://api.example.com/data') .then(res => res.json())</script>
三、工作原理解析
1. 基本流程
js
// Suspense 内部工作流程const handleAsyncContent = () => { try { // 1. 检测到异步内容 // 2. 显示 fallback 内容 // 3. 等待异步操作完成 // 4. 显示实际内容 } catch (error) { // 处理错误情况 }}
2. 状态管理
vue
interface SuspenseState { isResolving: boolean; // 是否正在解析 isHydrating: boolean; // 是否正在水合 async: boolean; // 是否存在异步依赖 error: Error | null; // 错误信息}
四、实战应用场景
1. 数据预加载
vue
<template> <Suspense> <template #default> <UserDashboard /> </template> <template #fallback> <LoadingSpinner /> </template> </Suspense></template> <script setup>// UserDashboard.vueconst UserDashboard = defineAsyncComponent({ loader: () => import('./UserDashboard.vue'), delay: 200, // 延迟显示 loading timeout: 3000 // 超时时间})</script>
2. 嵌套异步组件
vue
<template> <Suspense> <template #default> <div> <AsyncHeader /> <AsyncContent /> <AsyncFooter /> </div> </template> <template #fallback> <SkeletonLoader /> </template> </Suspense></template>
3. 错误处理
vue
<template> <Suspense @pending="onPending" @resolve="onResolve" @fallback="onFallback"> <template #default> <AsyncComponent /> </template> <template #fallback> <LoadingState /> </template> </Suspense></template> <script setup>const onPending = () => console.log('Loading started')const onResolve = () => console.log('Loading completed')const onFallback = () => console.log('Fallback rendered') // 错误边界处理onErrorCaptured((err) => { console.error('Async error:', err) return false})</script>
五、性能优化技巧
1. 延迟加载策略
vue
// 优化加载体验const AsyncComponent = defineAsyncComponent({ loader: () => import('./HeavyComponent.vue'), delay: 200, // 延迟显示 loading timeout: 3000, // 超时设置 suspensible: true, onError(error, retry, fail) { if (error.message.includes('fetch')) { // 重试加载 retry() } else { // 彻底失败 fail() } }})
2. 缓存处理
vue
// 缓存异步数据const cachedData = ref(null) const fetchData = async () => { if (cachedData.value) return cachedData.value const data = await fetch('/api/data') cachedData.value = await data.json() return cachedData.value}
六、最佳实践
1. 组件封装
vue
<!-- AsyncWrapper.vue --><template> <Suspense> <template #default> <slot></slot> </template> <template #fallback> <slot name="loading"> <DefaultLoading /> </slot> </template> </Suspense></template> <script setup>// 使用方式更简洁const DefaultLoading = () => import('./DefaultLoading.vue')</script>
2. 错误边界
vue
<template> <ErrorBoundary> <Suspense> <template #default> <AsyncContent /> </template> <template #fallback> <Loading /> </template> </Suspense> </ErrorBoundary></template>
总结
Suspense
是 Vue3
中处理异步加载的强大工具:
1.优点:
- 代码组织更清晰
- 处理异步更优雅
- 支持嵌套异步
- 错误处理更完善
2.使用建议:
- 合理使用
delay
避免闪烁 - 设置适当的超时时间
- 做好错误处理
- 考虑性能优化
3.记住,Suspense
不是万能的,它主要用于:
- 异步组件加载
- 异步数据获取
- 加载状态管理
合理使用 Suspense
,可以让你的代码更优雅,用户体验更好!
Preview
2
点个赞 ~
版权申明: © 本文著作权归YGHub所有,未经YGHub网授权许可,禁止第三方以任何形式转载和使用本文内容。
Related article
基于微信小程序实现图片压缩、裁剪、尺寸调整的实践总结
YGHub
2025-01-02
4
Vue3 作用域插槽,提升组件复用性的利器
YGHub
2024-12-03
1
Nuxt3 中使用 localStorage 的正确姿势
YGHub
2024-12-01
3
Vue3 子组件 defineExpose 暴露方法无效的三种常见场景及解决方案
YGHub
2024-11-24
7
Vue3 组件通信实战,实现跨组件数据更新
YGHub
2024-11-21
8