Go正则表达式处理多行文本:换行符匹配问题与解决方案 2024
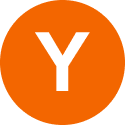
YGHub·2024-11-13·2·字数:374 字·阅读时间:2 分钟
在 Go 语言中处理多行文本的正则表达式时,换行符常常会导致意外的匹配结果。
问题描述
常见问题
go
text := `first linesecond linethird line` // 看似正确但可能失效的正则pattern := "first.*third"matched, _ := regexp.Match(pattern, []byte(text))fmt.Println(matched) // false
原因分析
- 默认情况下,
.
不匹配换行符 \n
和\r\n
的平台差异- 多行模式(multiline)与单行模式(singleline)的区别
解决方案
1. 使用 (?s)
标志(推荐)
go
// 启用单行模式(让 . 匹配换行符)pattern := `(?s)first.*third`matched, _ := regexp.Match(pattern, []byte(text))fmt.Println(matched) // true
2. 使用 [\s\S]
字符类
go
// 匹配任意字符(包括换行)pattern := `first[\s\S]*third`matched, _ := regexp.Match(pattern, []byte(text))fmt.Println(matched) // true
3. 结合多行模式 (?m)
go
// 处理多行文本时的行首行尾pattern := `(?m)^line\d$`matches := regexp.MustCompile(pattern).FindAllString(text, -1)
实战示例
1. 提取多行注释
go
func extractComments(code string) []string { pattern := `(?s)/\*.*?\*/` re := regexp.MustCompile(pattern) return re.FindAllString(code, -1)} // 测试code := `/* 这是一个 多行注释 */func main() { /* 另一个注释 */}`comments := extractComments(code)
2. 处理日志文件
go
func parseLogEntry(log string) []LogEntry { pattern := `(?m)^(\d{4}-\d{2}-\d{2})\s+(.*)$` re := regexp.MustCompile(pattern) matches := re.FindAllStringSubmatch(log, -1) var entries []LogEntry for _, match := range matches { entries = append(entries, LogEntry{ Date: match[1], Content: match[2], }) } return entries}
性能优化建议
1.预编译正则表达式
go
// 好的做法var commentRegex = regexp.MustCompile(`(?s)/\*.*?\*/`) func process(input string) { matches := commentRegex.FindAllString(input, -1) // ...}
2.使用合适的量词
go
// 避免回溯过多pattern := `(?s)/\*.*?\*/` // 使用非贪婪模式// 而不是pattern := `(?s)/\*.*\*/` // 贪婪模式可能导致性能问题
常见陷阱与注意事项
1. Windows 换行符
go
// 处理跨平台换行符pattern := `(?s)line1[\r\n]+line2`// 或者pattern := `(?s)line1\R+line2`
2. Unicode 支持
go
// 启用 Unicode 支持pattern := `(?s)(?U)first.*third`
3. 贪婪与非贪婪
go
// 非贪婪匹配pattern := `(?s)".*?"`// 贪婪匹配pattern := `(?s)".*"`
最佳实践总结
1.正则表达式标志的使用
(?s)
: 单行模式(?m)
: 多行模式(?i)
: 忽略大小写(?U)
: Unicode 支持
2.性能考虑
- 预编译正则表达式
- 使用非贪婪匹配
- 避免过度复杂的表达式
3.跨平台兼容
- 考虑不同的换行符
- 使用
\R
匹配通用换行
调试技巧
go
// 打印正则匹配过程debug := regexp.MustCompile(pattern)fmt.Printf("Pattern: %q\n", debug.String())fmt.Printf("Groups: %d\n", debug.NumSubexp())
总结
处理 Go 语言中的正则表达式换行符问题,关键在于:
- 理解
(?s)
标志的作用 - 正确处理跨平台换行符
- 选择合适的匹配模式
- 注意性能优化
参考资料
Preview
2
点个赞 ~
版权申明: © 本文著作权归YGHub所有,未经YGHub网授权许可,禁止第三方以任何形式转载和使用本文内容。
Related article
基于微信小程序实现图片压缩、裁剪、尺寸调整的实践总结
YGHub
2025-01-02
4
Vue3 作用域插槽,提升组件复用性的利器
YGHub
2024-12-03
1
Nuxt3 中使用 localStorage 的正确姿势
YGHub
2024-12-01
3
Vue3 子组件 defineExpose 暴露方法无效的三种常见场景及解决方案
YGHub
2024-11-24
7
Vue3 组件通信实战,实现跨组件数据更新
YGHub
2024-11-21
8