Vue3组件通信完全指南:8种方法对比与最佳实践 🔥
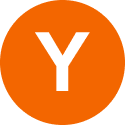
YGHub·2024-11-11·16·字数:613 字·阅读时间:3 分钟
在 Vue3 的开发中,组件间的通信是一个核心概念。本文将详细介绍 8 种主流的组件通信方法,并通过实例和最佳实践帮助你选择最适合的方案。
1. Props 和 Emits(父子组件通信)
最基础也是最常用的通信方式。
Props 向下传递
vue
<!-- 父组件 Parent.vue --><template> <child-component :message="message" :user-info="userInfo" /></template> <script setup>import { ref } from 'vue' const message = ref('Hello from parent')const userInfo = ref({ name: 'John', age: 30})</script> <!-- 子组件 Child.vue --><script setup>const props = defineProps({ message: String, userInfo: { type: Object, required: true }})</script>
Emits 向上传递
vue
<!-- 子组件 Child.vue --><template> <button @click="handleClick">更新</button></template> <script setup>const emit = defineEmits(['update', 'delete']) const handleClick = () => { emit('update', { status: 'success' })}</script>
最佳实践:
- Props 命名使用 kebab-case
- 明确定义 Props 类型和默认值
- Emits 事件名使用 kebab-case
- 避免过度使用,保持组件独立性
2. Provide/Inject(跨层级组件通信)
适用于需要跨多层组件传递数据的场景。
vue
<!-- 祖先组件 --><script setup>import { provide, ref } from 'vue' const theme = ref('dark')provide('theme', theme) // 提供更新方法provide('updateTheme', (newTheme) => { theme.value = newTheme})</script> <!-- 后代组件(任意层级) --><script setup>import { inject } from 'vue' const theme = inject('theme')const updateTheme = inject('updateTheme')</script>
最佳实践:
- 使用 Symbol 作为 key,避免命名冲突
- 提供默认值
- 考虑使用 readonly 防止意外修改
3. Pinia(全局状态管理)
适用于大型应用的状态管理。
ts
// stores/user.tsimport { defineStore } from 'pinia' export const useUserStore = defineStore('user', { state: () => ({ user: null, token: null }), actions: { async login(credentials) { // 登录逻辑 }, logout() { this.user = null this.token = null } }, getters: { isLoggedIn: (state) => !!state.token }}) // 组件中使用<script setup>import { useUserStore } from '@/stores/user' const userStore = useUserStore()</script>
最佳实践:
- 按功能模块拆分 store
- 使用 actions 处理异步操作
- 使用 getters 处理派生状态
4. EventBus(事件总线)
适用于非父子组件间的简单通信。
ts
// eventBus.tsimport mitt from 'mitt'export const emitter = mitt() // 组件 Aemitter.emit('userUpdated', { name: 'John' }) // 组件 Bemitter.on('userUpdated', (user) => { console.log(user)})
最佳实践:
- 谨慎使用,容易导致维护困难
- 及时清理事件监听
- 使用 TypeScript 定义事件类型
5. v-model(双向绑定)
适用于表单组件等需要双向数据流的场景。
vue
<!-- 父组件 --><template> <custom-input v-model="searchText" v-model:title="title" /></template> <!-- 子组件 --><script setup>defineProps(['modelValue', 'title'])defineEmits(['update:modelValue', 'update:title'])</script>
6. Refs(直接访问子组件)
适用于需要直接操作子组件的场景。
vue
<template> <child-component ref="childRef" /></template> <script setup>import { ref, onMounted } from 'vue' const childRef = ref() onMounted(() => { childRef.value.someMethod()})</script>
7. Slots(插槽)
适用于内容分发的场景。
vue
<!-- 父组件 --><template> <card-component> <template #header> <h2>卡片标题</h2> </template> <template #default> <p>卡片内容</p> </template> </card-component></template>
8. Vuex(传统状态管理)
虽然 Vue3 推荐使用 Pinia,但在某些场景下可能需要维护旧项目。
选择建议
根据场景选择合适的通信方式:
1.父子组件:Props/Emits
2.跨层级组件:Provide/Inject
3.全局状态:Pinia
4.表单组件:v-model
5.内容分发:Slots
总结
- 选择合适的通信方式对于应用的可维护性至关重要
- 避免过度使用全局状态和事件总线
- 保持组件的独立性和可复用性
- 合理使用 TypeScript 提高代码健壮性
参考资料
Preview
1
6
点个赞 ~
版权申明: © 本文著作权归YGHub所有,未经YGHub网授权许可,禁止第三方以任何形式转载和使用本文内容。
Related Article
Vue3组件库推荐:打造高效UI的6大选择
YGHub
2025-05-10
0
Vue3组件销毁时如何正确清理input焦点相关资源
YGHub
2025-02-14
0
Vue3中如何优雅地实现input自动聚焦(完整解决方案)
YGHub
2025-02-14
1
Vue3 作用域插槽,提升组件复用性的利器
YGHub
2024-12-03
3
Nuxt3 中使用 localStorage 的正确姿势
YGHub
2024-12-01
3
Vue3 子组件 defineExpose 暴露方法无效的三种常见场景及解决方案
YGHub
2024-11-24
7